Comp 151 Project 5
Using functions
Summary:
In this project you will practice everything from the last couple of weeks
to give you a final mid-semester project. You will be using more functions,
lists, working with files, selection, and repetition.
Due:
Friday March 8th at 11:59pm. (just before spring break)
Description:
My middle school children love silly text based emoji. You will get the
opportunity to write something for the middle-schooler in your life
- Your end result should look something like this:
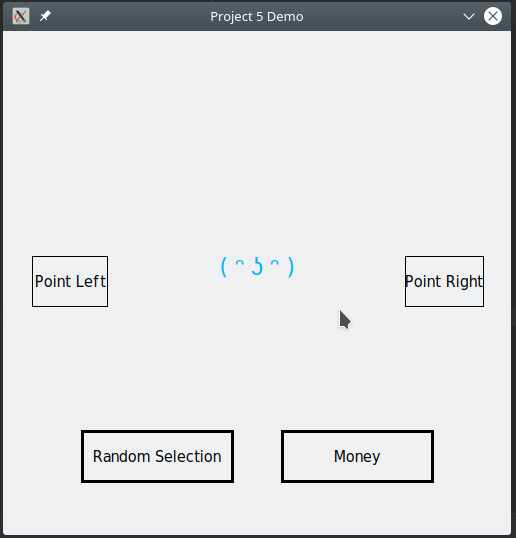
- When the left button is pressed it might look like this:
You need to have:
- a file with at least 10 text emoji, one per line
- Your main function.
- a function called load_emojis
- this function needs to open the emoji file, read all of the lines in
and load them into a list of strings
- This function must return the list of strings that you loaded.
- A function called is_clicked that I give you below.
- use that function to see if you user has pushed
- you need a graphics.Text object in the middle of the window that will
display the chosen emoticon.
- you need 4 rectangles to serve as 'buttons'
- they should each be labeled to tell the user what that button does.
- one should be on the left and should put an emoji that indicates
left in some way
- one should be on the right and should put an emoji that indicates
right in some way when the rectangle is clicked.
- Two buttons should be on the bottom
- one should tell me what it does and put up an emoticon that
matches that
- the other should indicate that it will put up a random emoticon
and do so when pressed.
To make your life a little easier, you can use the function below to help
you determine if one of the fake buttons is pushed (and do nothing if the
mouse is clicked outside of those fake buttons).
def is_clicked(rect:graphics.Rectangle, point:graphics.Point):
xloc = point.getX()
yloc = point.getY()
button_width = abs(rect.getP1().getX() - rect.getP2().getX())
button_height = abs(rect.getP1().getY() - rect.getP2().getY())
center = rect.getCenter()
if xloc >= center.getX()- button_width/2 and xloc <= center.getX() + button_width/2 and \
yloc >= center.getY() - button_height/2 and yloc <= center.getY() + button_height/2:
return True
else:
return False
Just paste the function in your code above your def main(): line.
For the example below, assume that click_loc is initialized as follows:
click_loc = window.getMouse()
and random_rect is the graphics.Rectangle object that I used to
draw the "Random Selection" 'button' up above. Then can use this
is_clicked something like the following:
if is_clicked(random_rect, click_loc):
Additional requirements:
To help me identify your work, make the name of your program file
yourFirstInitialLastNameLab1.py
(so mine would be JSantoreLab1.py)
And put the following at the beginning of
the program as a comment:
- Your name (There
will be credit assigned for this stuff)
- Anything that was left undone (we are
getting deep enough in the semester that some of you will not plan
enough time and will not get it all done, tell me here)
- Anything that I need to know to run the
program correctly.
Project Submission
Submit your project via blackboard. For this fifth project you need to
submit the entire zipped project folder because I need your image and your
graphics.py and your text file of emoji along with your code..