Comp 152 Project 2
Due: Wednesday Feb 19th at
Midnight (note this is the Monday Schedule of
classes at BSU)
Summary and goals:
In this project you will have another chance to use ArrayLists since my
experience is that the first project just gets you started and a second one
really solidifies your understanding of ArrayList collections. You will also
use UML to help
develop your project more
readily.
Details:
Write a program with three classes and the enum as seen in the UML diagram
below.
This program will simulate the electoral college in presidential election
years. The 2010 census gave us the 2012 electoral college numbers which we
will be using for the next couple of elections.
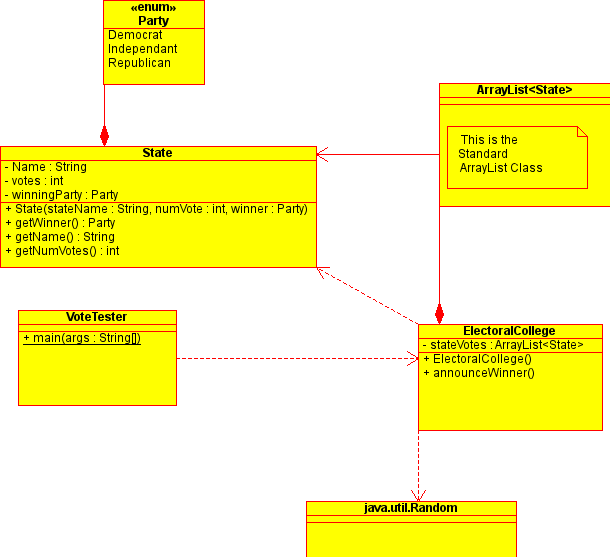
Implementation Specification:
Party: This is an enumerated type
with three possible values (in alphabetical order): Democrat, Independent,
Republican
I'll introduce these in class for those who didn't have
enums in comp151.
State: This class encapsulates a state in the electoral college.
It has three instance variables which should be initialized by the
three parameters to the constructors. The other three methods are all
accessors which retrieve the value of the associated instance variable.
ArrayList<State>: this is
the standard ArrayList in the Java.util package.
Electorial College:
In the constructor
first create the stateVotes instance variable. Next open the file Electoral2012.txt
and read out the state names and the number of electoral votes for each
state. Use a java.util.Random object to assign the state to one of the
three parties for the Party Enum. Lets say that Democrats and
Republicans should each have a 2 in 5 chance of winning. The
independant should have a one in 5 chance of winning. Create the
resulting State object and store it in stateVotes. Do this for all 50
states (plus the district of Columbia).
In announceWinner() look through stateVotes and add up the total
electoral votes for each of the three parties. Print out the total
electoral votes for each party and if any party has more than the 270
needed to win, announce that party as the winner - otherwise annouce
that Congress will have to make the choice. Finally, print the
individual winner of each state.
VoteTester: This class
contains only the main method. The main method should create an
ElectoralCollege object and then call announceWinner on that object.
You must have all of the methods and instance variables specified in
the UML. You may have any additional methods that you wish to make the
project easier to implement.
Readme:
You also need to have a readme.txt file in your folder which contains
- your name
- a brief (one paragraph) description of how
you implemented the project, in particular, how did you decide to keep
everything ordered?
- anything that is left undone in the project.
Submission:
As before, you need to submit using moodle: