Comp 152 Project 3:
Inheritance
Due: Monday Oct 21 by the end of the
day. (your midterm is later that week)
Project:
You will write a program which will demonstrate inheritance using some
simple classes which might form the backend of a 2d game with bit more
work. Implement the UML found below along with a few extra methods found in
the details below the UML:
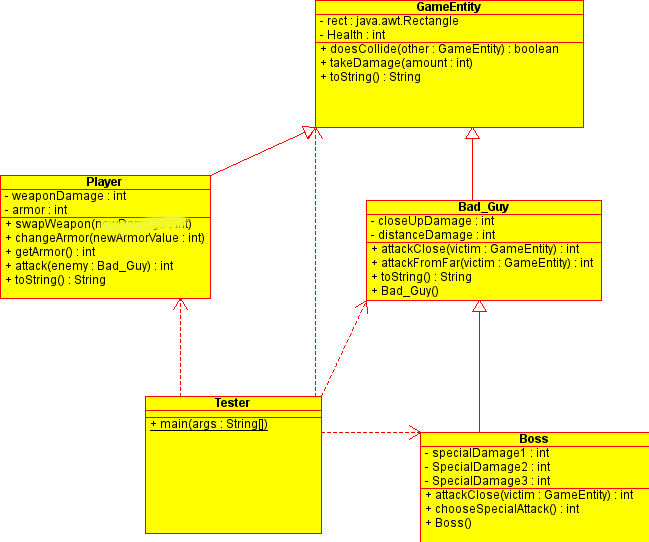
Methods:
The methods in the UML class diagram above should be implemented as follows:
- GameEntity Class
- doesCollide: should return true if the rect for this one overlaps
the rect for the other GameEntity (hint: see the
java.awt.Rectangle documentation for a method to do this for
you)
- takeDamange: reduces health by amount
- toString should print out the entity and its health
- Player
- add A constructor (not in the UML above). The constructor should
read from a file
- a set (at least 4) of weapon names and damage values. Store this
in an instance variable whose name you select (it is not in the
diagram so you can choose your own name and type. Make sure that I
can add a new weapon/damage combo and your code will still work for
all of them.
- start with the usual file loading code - then to help you out
consider the following:
- swapWeapon:
- display the weapon names that you loaded in the constructor and
let the user pick one. Then set weapon damage to the damage value
for that weapon.
- changeArmor: should set armor to newArmorValue
- getArmor: should return armor
- attack : should call takeDamage on the enemy
- takeDamage: should reduce the damage by (armor/1000) percent and
then call the parent class takeDamage
- toString: standard toString then call parent class toString
- Bad_Guy:
- attackClose should call takeDamage on victim passing closeUpDamage
as the parameter
- attackFromFar should call takeDamage on victim passing
distanceDamage as the parameter
- the Constructor should initialize the two instance variables to some
int between 100 and 200 of your choice
- Boss
- Constructor should set the three special damages to three values
from 100 to 500 (you choose how)
- attackClose should call chooseSpecialAttack and then us its return
value as a parameter to call takeDamage on victim
- chooseSpecialAttack should randomly choose (using a java.util.Random
object) a value from one to three and return that specialDamage value
as its return value (for example if you randomly choose two then
return SpecialDamage2)
- Tester
- main: this is the driver. It should test the various classes as
follows
- create a player, a bad guy and a boss.
- Repeat the rest below:
- ask the user if they wish to keep playing
- if so:
- ask the user what the players armor should be
- changeArmor to that value
- call swapWeapon to let the player select a weapon
- set the damage appropriately
- have the Bad_Guy attackClose on the player
- have the Player attack the Bad_Guy
- print out the each of your three objects (you'll need to use
your toString)
- Have the player attack the Boss
- have the boss attack the player
- print out the each of your three objects (you'll need to use
your toString) again
Extra requirements for the project
Comment your code.
Include comments at the top of your Tester class
your name
Anything you didn't get done
Submission:
upload to github and make me a collaborator as before.